
Turtle
The parameters in the Turtle and Values tabs are meant for advanced users with programming experience (these tabs contain an entire L-System). Those of you with little or no programming experience should, however, not be scared off. In the following are several variable examples.
Make sure you first set Mode to Turtle.
Brief introduction to L-Systems
In the following pages, the L(indenmayer)-Systems are briefly explained. Additional books or online resources are available that offer an in-depth explanation of these systems (the pdf document "The Algorithmic Beauty of Plants" from Przemyslaw Prusinkiewicz and Astrid Lindenmayer is based on use with Cinema 4D and can be downloaded at http://algorithmicbotany.org/papers#abop).
L-Systems are usually used for simulating plant growth and similar effects. Combined with MoGraph, these can be used to create spectacular growth simulations by placing a MoSpline object into a Sweep object, thus creating a renderable object (Splines can be rendered directly using Sketch and Toon or HAIR).
Simply put, an L-System executes a series of commands that define how and where new branches should grow. It is a self-producing system that can replace existing branches with new ones.
The basic principles are fairly simple and are based on a system called "Turtle System". A (virtual) turtle is sent on its way via simple commands. This path is represented by a Spline.
The 3 most important commands are:
- A simple F, which simply means "Go one step Forwards". For a MoSpline it means "Extend the Spline by one unit (in the object’s Z direction)"
- +: Rotate right
- -: Rotate left
If you enter the sequence F++F++F in the Premise field it translates to: "Take one step Forward; turn twice to the right; take another step Forward; turn twice to the right; take another step Forward."
How big each step is and how large the angle of rotation is can be defined in the Values tab via the Default Movement and Default Angle values, respectively. Or, as described below, by placing them in parenthesis. If Default Angle is set to 60° a perfect triangle will result for our example:
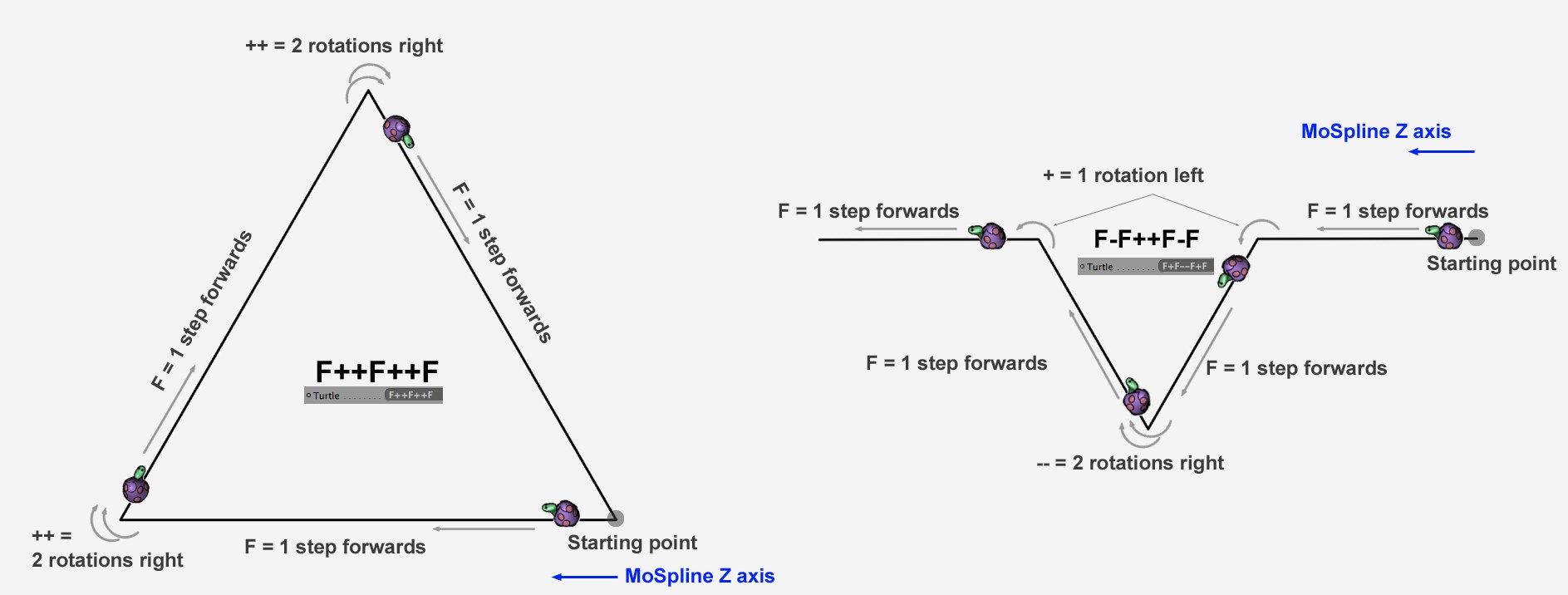
This lets you create branch-like shapes. A very interesting function of L-Systems is its integrated replacement system with which individual branches can be replaced by others. This can also be done recursively, which lets you create very complex branching with simple command codes. If this sounds confusing, the following example can shed some more light on what’s involved:
So far you have only entered single-line command codes that were executed only once. Now enter the following command code into the respective fields:
Premise field: F++F++F
Rules field: F = F-F++F-F
As you can see, the Rules code line contains an assignment (in the following referred to as Rule) in which "F" is assigned several symbols that in turn will (internally) be inserted into the Premise line of code.
If written out in its entirety, the command line code would read as follows (replace the F in the Premise line of code with the code following the "F" in the Rules line of code):
F-F++F-F++F-F++F-F++F-F++F-F
This represents the second shape from left in the image below. Since each F in the Premise code is replaced by the Rule, each straight line will be replaced by the more complex line.
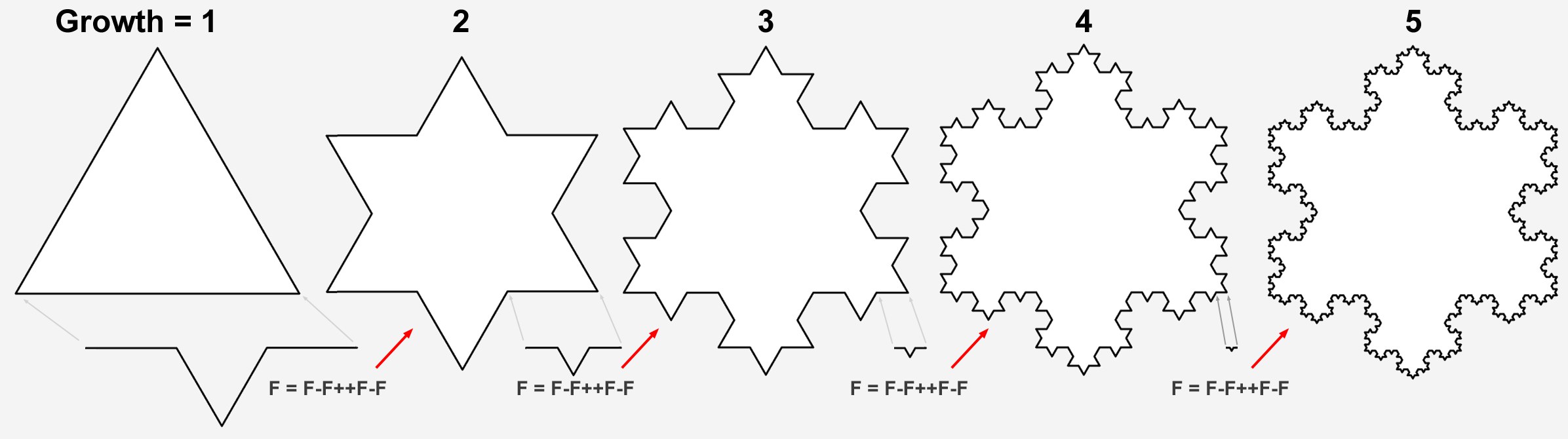
And this replacement process can be repeated indefinitely (well, not quite, since your computer will probably throw in the towel after the exponentially increasing complexity of the Splines starts to grow … ). How often this replacement process is repeated is defined by the Growth value, which replaces each F with the Rules code. Incidentally, fractals work the same way.
Of course placeholders can be used as well so that not necessarily each F has to be used. After all, this would be unrealistic for growing formations - growth in length does not always occur only at the end of branches, etc.
For this you can use placeholders (letters that do not represent any Turtle command) such as A, B, C, D, (but NOT "F" because it is the default "one unit forward command").
Type the following into the respective fields:
Premise: FA
Rules: A=+F-FA

Since the Rule ends with an A, an increasing Growth value will result in the formation growing endlessly (Default Angle is set to 90°).
The code we have used so far has consisted of a single, uninterrupted line of code. However, L-Systems first become interesting when real branches are created, i.e., new lines that branch from existing lines. And this can be achieved by using square brackets ([ ]). The command sequence within a square bracket is viewed as a separate branch (and creates a new Turtle). After the branch has been created the turtle will return to the starting point prior to the square brackets and continue with the command sequence following the square brackets.
Type the following into the Premise field:
F[+F][-F[-F]F]F[+F][-F]
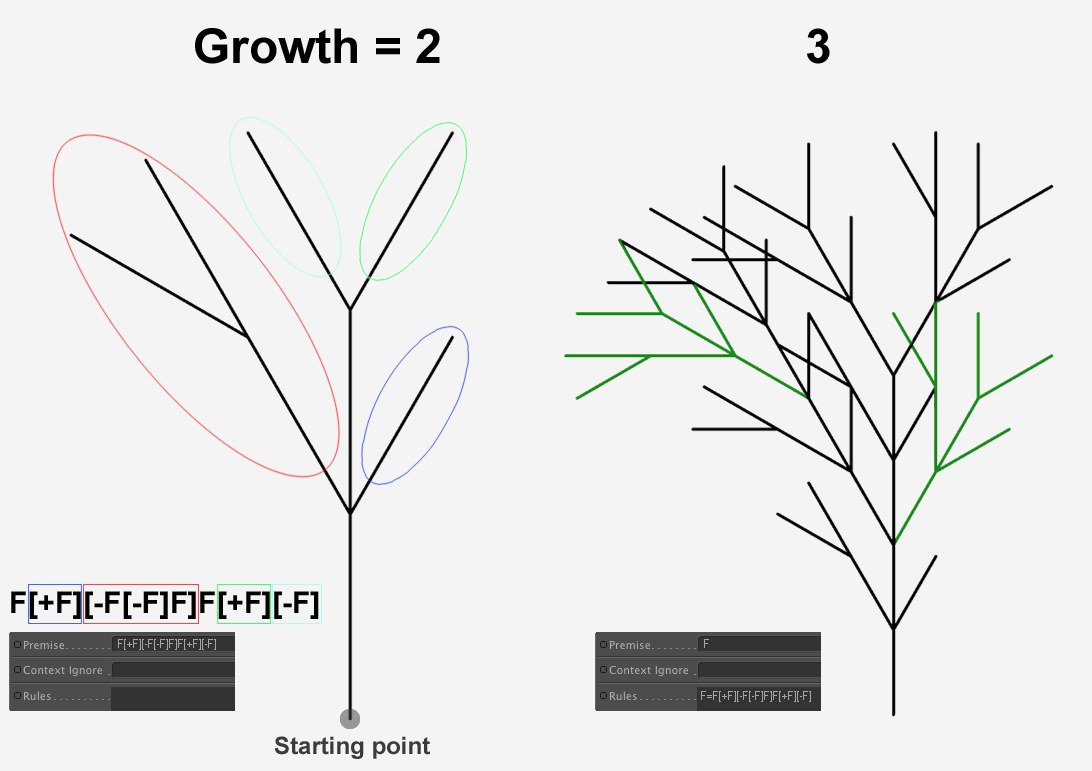
If you now enter F into the Premise field and the above code (F=F[+F][-F[-F]F]F[+F][-F] ) into the Rules field, each F will be replaced by the braches at the left of the image. Increasing the Growth value will let the branch continue to grow because each straight line will be perpetually replaced by a new branch. If you look closely you will see the branch pattern at the left repeated in the tree at the right (highlighted in green).
This is an easy method of creating complex plant-like branching:
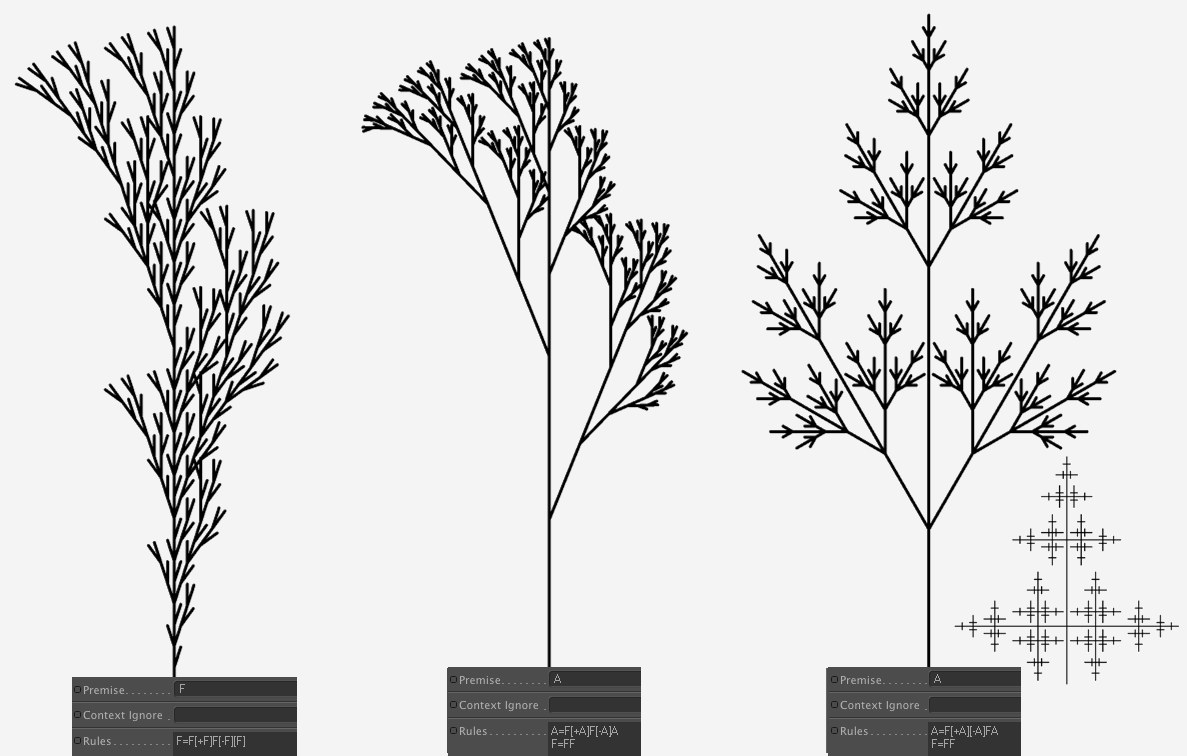
Until now we have generated everything on a flat plane. Since branching doesn’t only take place on a single plane but in every direction, command symbols exist that also makes this possible.
These are (a degree value can be added in brackets after the command symbols):
& or ^: rotate around the Turtle’s transverse axis
\ or /: rotate around the Turtle’s longitudal axis
Type the following into the Premise field: F&F\ (90)^F+F:
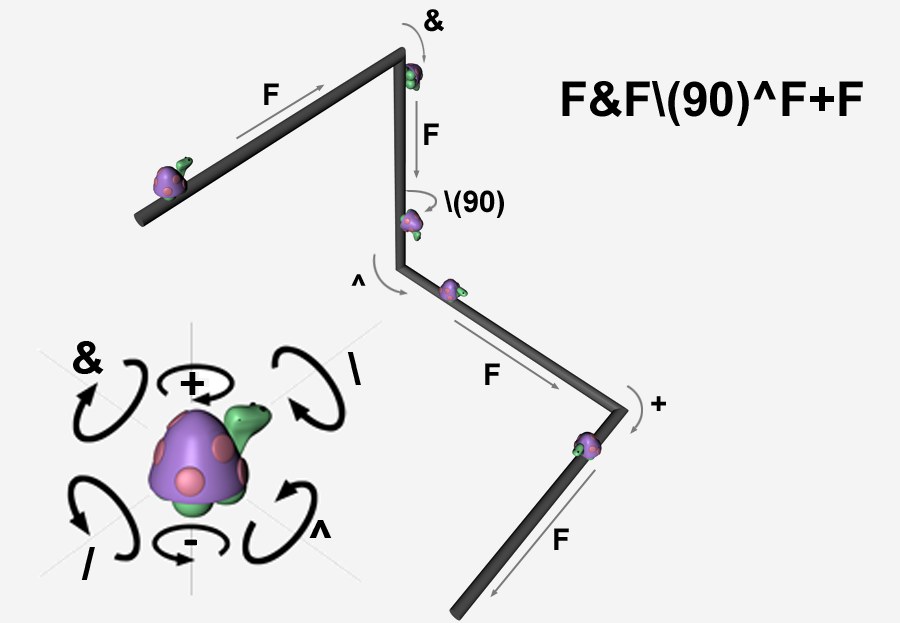
The result is the Spline pictured above. The turtle moves forward (F), rotates at a right-angle around its transverse axis (&, Default Angle is set to 90°), moves forward again (F), rotates 90° around its longitudal axis (\ (90)), then 90° around its transverse axis (^), moves forward again (F), turns right (+) and finally moves forward again (F).
If you use this method to create branched spatial formations, real bushes and trees can be created.
User Data
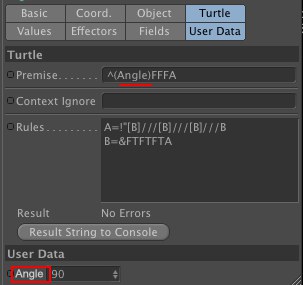
You can use the User Data as variables in a sequence of symbols. In the image above an Angle parameter was added to the User Data that was then substituted in parenthesis for ^ (turtle rotates around transverse axis) in the Premise field.
Special characters and spaces should not be included in the variable names. Underscores are recognized (e.g., "second_length") as is capitalization.
Formulas
Formulas, including defined variables (e.g., F(_growth*2), can be used instead of normal digits. The following are available:
_total | The total number of commands in the current string (see below) |
_index | The current command index in the string (see below( |
_growth | The current growth level |
_level | The current command level (if assigned, see below) |
_x, _y, _z | The current Turtle position in space. |
_arg1, _arg2, _arg3, _arg4 | The first, second, third and fourth argument values (e.g., from F(1,2,3,4)) |
_age | The current command age (represents _growth - _level) beginning with 0.0. |
_length | The current arc length between Turtle and MoSpline origins. |
_move | The current default scale length. |
_tropism | The current Tropism. |
_scale | The current default scale. |
_rotate | The current default angle. |
_rnd, random | A random number between -1.0 and +1.0 |
Example "Total/Index"
Let’s say you have the string F(_index)F(_index), which consists of two commands. The string performs the same function as F(1)F(2) (one unit forwards then two units forward). For example, since "_total" is the total number of commands, a spiral can be created with the following command:
Premise: FA
Rule: A=+(20*_index/_total)FA
Example "_RND"
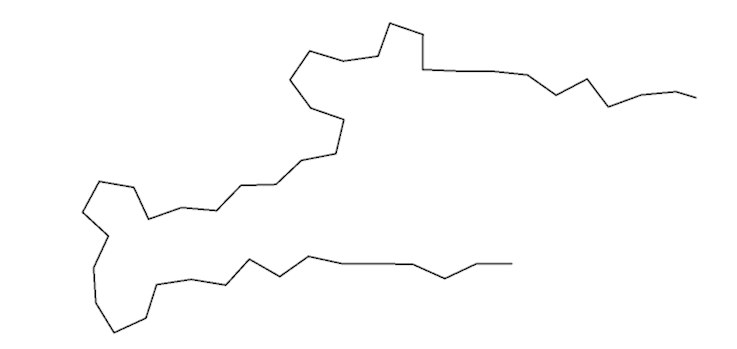
The following command:
Startstring: A
Regel: A=+(_rnd*90)FA
The, _rnd*90’ command will ascertain a random angle between -90 and +90 for each growth spurt, i.e., a "wobbly" line with random rotations will result.
Example "_level"
Let’s say you have the following command:
Premise: A
Rule: A=FA
The following level values will result (shown here in parentheses for demonstration purposes - these will NOT actually be shown):
Growth = 0 : A(0)
Growth = 1 : F(1)A(1)
Growth = 2 : F(1)F(2)A(2)
Growth = 3 : F(1)F(2)F(3)A(3)
Now that you know how the "_index" numbering is done, take a look at the following command:
Premise: FA
Rule: A=+F(_level*10)A
… creates the following spiral with Default Angle set to 90°:
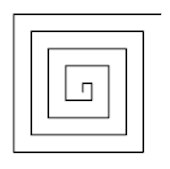
Example "_arg"
Just as you can define F(a,b,c)=F(a*2,b*2,c*2) to set parameters for Turtle commands (in this example F) you can also use the "_arg" command. These don’t have to be evaluated at the beginning. F=F (_arg1*2,_arg2*2,_arg3*2) does the same as the command above.
Advanced syntax variations
The following syntax is also possible:
Premise: F(2)F(1,2)F(2,3)
Rule : F(a,b) = F(a*2,b*2)
All Fs will be replaced and wherever "a" or "b" appear within the Rule existing F arguments will be handled as follows:
F(2*2,1*2)F(1*2,2*2)F(2*2,3*2) which logically equates to F(4,2)F(2,4)F(4,6).
Rule constructs like the following are also possible:
F(a,b):(a>10)=F(a,b)+F(a/2)
This Rule will, for example. only be applied if the value of the first argument is greater than 10.
In the following example both syntax variations above were combined and produce an interesting effect:
Example "fruits"

In the example above, several branches grow simultaneously. When one branch has finished growing a sphere will be placed at the end of the branch (requires the command J, see example: Cloner Object).
Note that "B(6)" is defined in the first Rule and that "B(h-1)" reduces the argument by 1 in Rule 2 until "h=0" finally sets the sphere in Rule 3.
Take a look at the console to see the entire string (but first click on the Result String to Console button).
Further details regarding formulas can be found in the attachment.
Enter the premise here. This is most often a single-letter command (see Rules) or a placeholder to which content is added in the Rule field. In the following pages you will find numerous examples of how strings can look.
Here you can define a series of symbols (commands) that will be ignored by context rules. Example:
You have the following constellation:
Premise: A+B+C+D+E+F
Rules: A<B=C
F(a,b,c) | Moves the Turtle forwards and optionally draws lines along its length (a), for scale (b) and/or subdivision (c). |
f(a) | Moves the Turtle forwards and NO line will be drawn (i.e. a Spline segment will be ended) "a" is the optional length value. |
H(a,b,c) | Moves the Turtle forwards and optionally draws a line half as long as its length (a), its scale (b) and/or its subdivision (c) |
h(a) | Moves the Turtle forwards and NO line will be drawn (i.e. a Spline segment will be ended) "a" is the optional half length value. |
M(x,y,z) | Moves and draws a line. The Turtle will move within local coordinates (x,y,z) but will itself not rotate. |
m(x,y,z) | Turtle moves forward but does not draw a line. The Turtle moves within local coordinates (x,y,z) but will itself not ,rotate. |
G(a) | Turtle moves forward but does not draw a line and does not end a Spline segment. "a" is the optional length value. |
R | Resets the Turtle back to the segment starting point, resets the rotation and draws a line (in a MoSplineTurtle a segment is always contained in [ ]). |
r | Resets the Turtle back to the segment starting point, resets the rotation and does not draw a line (in a MoSpline Turtle a segment is always contained in [ ]). |
P(x,y,z) | Places the Turtle at these coordinates and draws a line. |
p(x,y,z) | Places the Turtle at these coordinates and does not draw a line. |
-(a) | Rotates the Turtle counterclockwise around the vertical axis. "a" is the optional angle value. |
+(a) | Rotates the Turtle clockwise around the vertical axis. "a" is the optional angle value. |
&(a) | Rotates the Turtle forwards around the transversal axis "a" is the optional angle value. |
^(a) | Rotates the Turtle backwards around the transversal axis. "a" is the optional angle value. |
/(a) | Rotates the Turtle clockwise around the center axis. "a" is the optional angle value. |
\(a) | Rotates the Turtle counterclockwise around the center axis. "a" is the optional angle value. |
[ | New branch = new Turtle |
] | Branch ends |
{ | Polygon begin (see example below: "Polygon creation") |
} | Polygon end |
. | (Dot - Full Stop) Adds a polygon point - within curly brackets for polygons: e.g. = { . -f . +f . +f . +f } |
| | Rotates the Turtle 180' around the vertical axis. |
* | Rotates the Turtle 180' around the center axis . |
% | Prunes the branch at this point - everything will be ignored though to the end of the close bracket: ] see example "Prune branch". |
"(a) | Multiplies the length by the optional "a" value tor each generation (see example below .Example: Multiply/divide). |
!(a) | Multiplies the scale by the optional "a" value for each generation (MoSpline diameter when used in conjunction with Sweep object). |
;(a) | Multiplies the angle by the optional value "a" for each generation. |
_(a) | Divides the length by the optional value "a" for each generation. |
?(a) | Divides the scale by the optional value "a" for each generation (MoSpline diameter when used in conjunction with Sweep object). |
@(a) | Divides the angle by the optional value "a" for each generation. |
T(a) | Adds Tropism (e.g. FTFTF), Which means that each Spline segment will curve slightly in the direction of the global Y axis. Any particle modifiers present will be evaluated. |
$(x,y,z) | Displays the end of an Up Vector and orients the Turtle accordingly. Define a vector in whose direction the Turtle should face. |
I(a,r,g,b) | (First character string begins with a capitol "i") |
J(a,r,g,b) | |
K(a,r,g,b) | |
L(a,r,g,b) | For the above "I, J, K, and L", any number of clones generated by a Cloner object can be arranged along the Turtle Spline. "r,g,b" represents the color of the clone . "a" is the index (i.e. the number that represents the order in which an object is arranged below a Cloner. see "Index" below). I, J, K, L represent the respective Groups in the Cloner object's Mode parameter, "Group 1", "Group 2", "Group 3", "Group 4". An example is given below under "Example: Cloner object". |
Example, creating polygons’
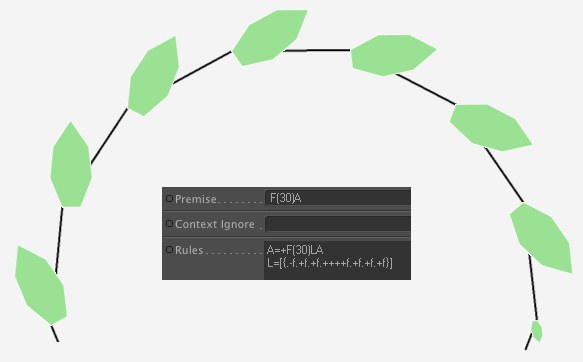
Take a look at the second line of the Rule field:
L=[{.-f.+f.+f.++++f.+f.+f.+f}]
Between the curly brackets (these define a polygon), a specific number of polygon points is defined (each begins with a ".") between which a polygon is created. Because the curly brackets are contained within square brackets, the turtle will be reset to its point prior to creating the leaf after each leaf has been created.
The entire leaf will be assigned to an "L" and the Rule in the line above will create a complete leaf wherever an "L" exists.
Note that the MoSpline can produce either a Spline OR a polygon. If you want to produce both simultaneously, use the Destination Spline.
Example "multiply/divide"

If you use a self-replacing sequence of symbols in the Rules field (e.g., A=F+BA), a multiplier or divisor (", !, ;, _, ?, @) can be used to increase or decrease the value of each new Generator.
Example "cutting a branch"
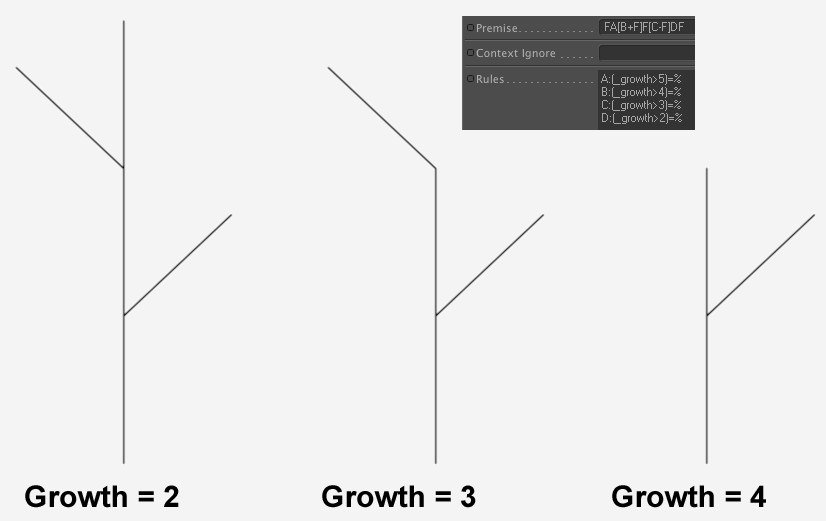
If you take a look at this Rule you will see that the placeholders A, B, C and D have been added to the premise. For various Growth values, the Rules now define the replacement of each placeholder with a % value, which means that the following symbols will be cut off at that point on (and per) each branch.
Example "Cloner object"

Note how J and K are used in the Rule (these could be replaced with (1,1,1,1) and (1,1,1,1), respectively, and if the parentheses argument were omitted each would use the standard value) that refers to the clones of a Cloner object. The mode must be set accordingly for both Cloner objects:
I(1,1,1,1) reflects Group 1
J(1,1,1,1) reflects Group 2
K(1,1,1,1) reflects Group 3
L(1,1,1,1) reflects Group 4
Note that one Rule
Premise: FA
Rules: A=FX
X=J(1,1,1,1)
may function but the clones don’t grow steplessly but are simply displayed abruptly.
Example "index"

A Cloner object’s Child objects are numbered sequentially (starting with "0"). If, for example, you define A=FJ(2,1,1,1)A (the RGB values can be omitted), the Cloner object will only use the object with the index "2" (left of the sphere). If you use A=J(rnd(2))A on the right side, a clone will be placed randomly in accordance with a number between "0" and "2" that is also chosen randomly.
Example "random growth"

This Rule in combination with varied User Data values for Limit1 and Limit2 (together, these values should equal 100%) can be used to create differing but very similar "plants" (Stochastic L-Systems. The conditions (rnd(1)<) or (rnd(1)>) determine randomly which of the 3 Rules will be applied (each with a new random selection for each new Generator, i.e., larger Growth parameter).
Example "context rules"
Rules can also be written in the following form:
Premise: ABC
Rule: A<B=C
Result (if Growth > 1): ACC
So what will happen? This depends on the sequence within the result string. The Rule "A<B=C" searches for instances within the string where a "B" directly follows an "A" and replaces the "B" by a "C".
If, on the other hand, you enter "A>B=C", the "A" to the left of a "B" will replaced by "C" (the result would be "CBC").
For the Rule "AB=C" it cannot be determined which symbol should be replaced by "C". In this case both will be replaced by "C" (the result would be "CCC").
Displays syntax errors.
Clicking on this button will display the symbol sequence currently in effect, taking into account all Rules and the Growth parameter, in the Console. Such a sequence can, for example, look like this (this is the sequence of symbols from the fruits example with Growth set to 4):
